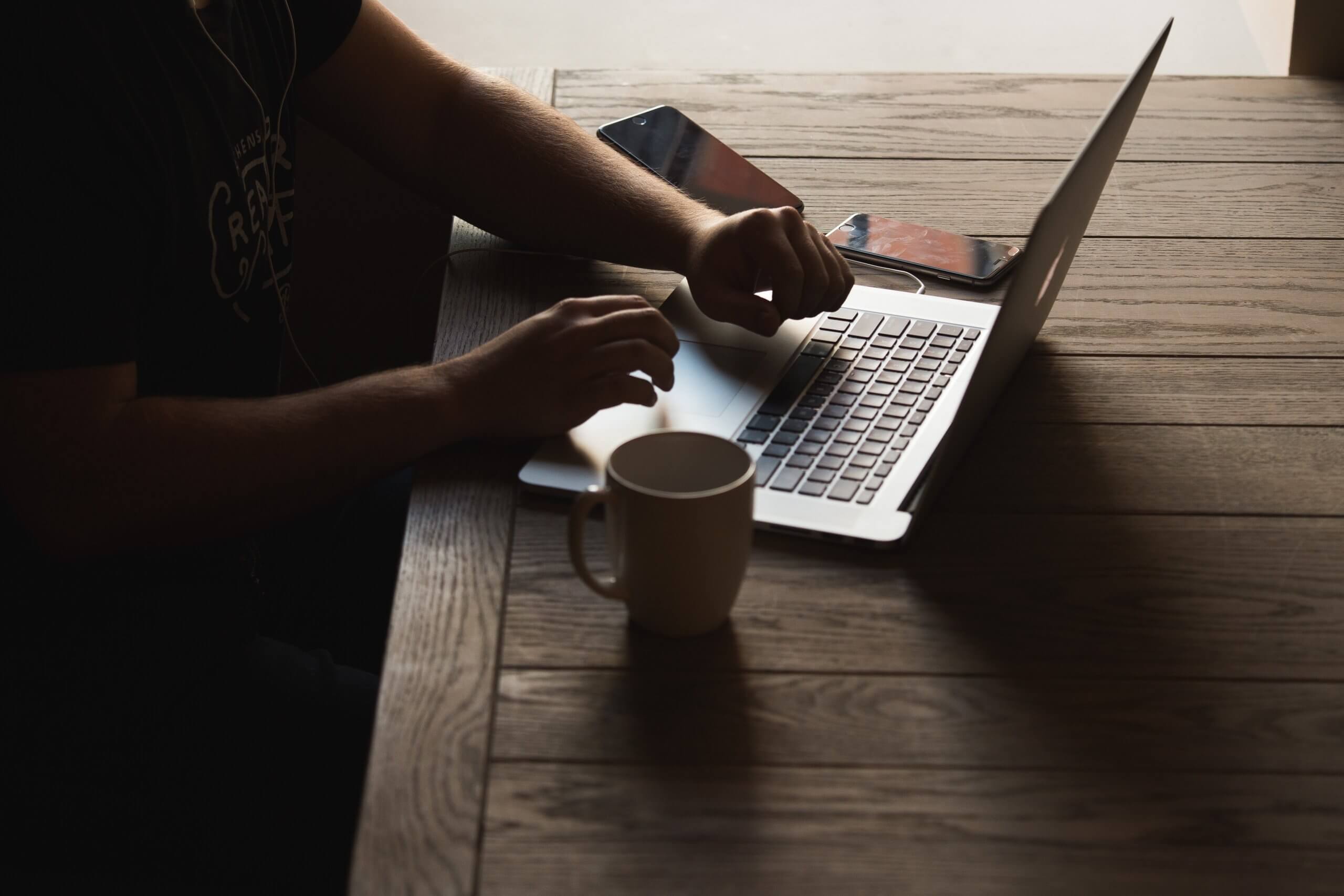
How to Edit Metadata of EPUB Using Python
EPUB files are a popular format for eBooks due to their flexibility and compatibility with various e-readers. Metadata in an EPUB file includes information such as the title, author, publisher, and description, which is crucial for categorizing and displaying the book correctly. Python provides a straightforward way to edit EPUB metadata using libraries like zipfile and lxml. Here’s a detailed guide on how you can modify EPUB metadata programmatically.
Understanding the Structure of an EPUB File
An EPUB file is essentially a compressed archive containing multiple files and directories. These include content files, images, stylesheets, and metadata. The metadata is typically stored in a file called content.opf, located in the OEBPS directory of the EPUB archive. This file adheres to the Open Packaging Format (OPF) standard and uses XML to structure the metadata.
To edit the metadata, you need to extract the content.opf file, modify its contents, and then repackage the EPUB file. Python makes this process efficient and straightforward.
Setting Up Your Python Environment
Before you begin, ensure that you have Python installed on your system. You will also need the lxml library for XML parsing and editing. If you don’t already have it installed, you can do so using pip:
pip install lxml
Extracting and Modifying Metadata
To edit the metadata of an EPUB file, first extract the content.opf file. Use Python’s zipfile module to access the EPUB file as a compressed archive. Locate the content.opf file and parse its XML content using lxml.
Once the file is parsed, you can navigate the XML tree to locate and edit the desired metadata elements. For instance, you can change the title, author, or description by updating the corresponding tags.
Saving and Repackaging the EPUB File
After modifying the metadata, save the updated content.opf file back into the EPUB archive. This requires replacing the old content.opf file with the modified version. Python’s zipfile module can handle this process, ensuring that the integrity of the EPUB file is maintained.
Example Code
Below is an example of how to edit the metadata of an EPUB file using Python:
import zipfile
import lxml.etree as ET
# Path to the EPUB file
epub_path = “example.epub”
# Open the EPUB file as a zip archive
with zipfile.ZipFile(epub_path, ‘r’) as zip_ref:
zip_ref.extractall(“temp_epub”)
# Path to the content.opf file
opf_path = “temp_epub/OEBPS/content.opf”
# Parse the content.opf file
with open(opf_path, ‘r’, encoding=’utf-8′) as file:
tree = ET.parse(file)
root = tree.getroot()
# Update the title metadata
namespace = {‘dc’: ‘http://purl.org/dc/elements/1.1/’}
title_element = root.find(“.//dc:title”, namespaces=namespace)
if title_element is not None:
title_element.text = “New Title”
# Save the modified content.opf file
with open(opf_path, ‘wb’) as file:
tree.write(file, encoding=’utf-8′, xml_declaration=True)
# Repack the EPUB file
with zipfile.ZipFile(“modified_example.epub”, ‘w’) as zip_ref:
for foldername, subfolders, filenames in os.walk(“temp_epub”):
for filename in filenames:
filepath = os.path.join(foldername, filename)
zip_ref.write(filepath, os.path.relpath(filepath, “temp_epub”))
print(“Metadata updated successfully.”)
Additional Considerations
While editing EPUB metadata is relatively straightforward, ensure that you do not inadvertently alter other parts of the file structure. Always maintain a backup of the original file before making changes. Test the modified EPUB file on multiple devices to confirm that the metadata is displayed correctly.
Editing the metadata of an EPUB file using Python is a practical and efficient way to manage eBooks. By leveraging libraries like zipfile and lxml, you can automate the process of modifying titles, authors, and other metadata fields. Whether you’re managing a personal library or preparing eBooks for distribution, Python provides the tools to make the task seamless and reliable.