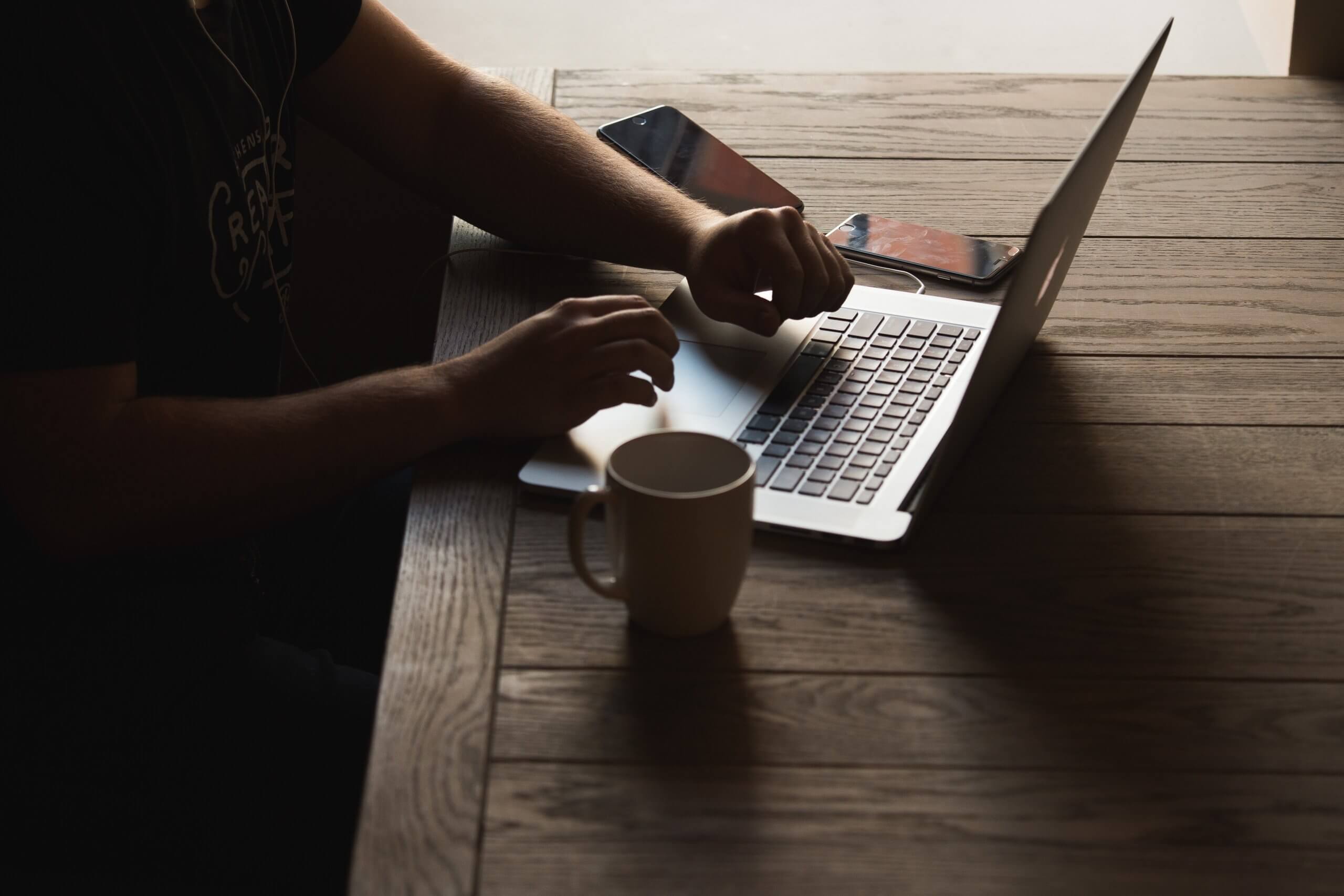
How to Pass Parameters into URL for Python Request
When working with APIs or web scraping in Python, one of the most common tasks is passing parameters into a URL for making HTTP requests. Whether you’re fetching data from an API or interacting with a web application, understanding how to properly pass parameters into your URL is crucial. This article will walk you through the essentials of using Python’s requests library for this purpose, providing tips, examples, and insights to make your experience as smooth as possible.
Understanding URL Parameters
A URL can contain parameters in the form of a query string. These parameters usually follow a question mark (?
) in the URL and are written as key-value pairs separated by an ampersand (&
), for example:
https://api.example.com/resource?key1=value1&key2=value2
The key represents the parameter name, while the value represents the data being sent. Handling these parameters effectively is critical when working with requests via Python.
Using Python’s requests Library
Python’s requests library is a powerful tool that allows you to interact with web services. To pass parameters into a URL, the most common approach is to use the params
argument in the requests.get()
method.
Basic Example
Here’s a simple example demonstrating how to pass parameters:
import requests
# URL of the API or resource
url = "https://api.example.com/resource"
# Parameters to pass
parameters = {
"key1": "value1",
"key2": "value2"
}
# Make the GET request with parameters
response = requests.get(url, params=parameters)
# Print the final URL and the response
print("Final URL:", response.url)
print("Response:", response.text)
In this snippet, the params
dictionary contains the key-value pairs to pass into the URL. The requests library automatically encodes these parameters and appends them to the base URL.
Why Use Parameters?
Passing parameters into a URL simplifies the process of customizing API requests. Here are a few scenarios where parameters are commonly used:
- Filtering Data: Access only the specific data you need, such as filtering by date, location, or category.
- Paginating Results: Fetch large datasets in smaller chunks using offset and limit parameters.
- Authentication: Include API keys or tokens directly in the request URL.
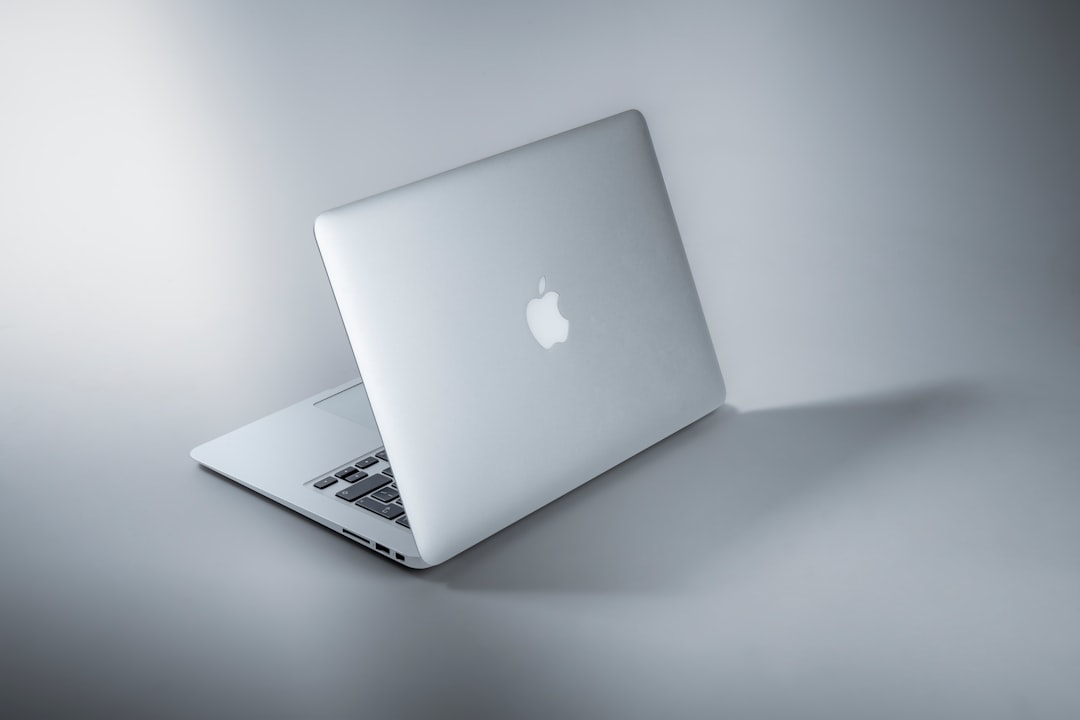
Tips for Handling Parameters
For a seamless experience, consider the following tips when passing parameters into URLs:
- Use a Dictionary for Parameters: Always define your parameters in a dictionary. This makes the code cleaner and allows the requests library to handle encoding for you.
- Double-Check Special Characters: Some parameters (such as those containing spaces or symbols) require proper URL encoding. Luckily, the requests library takes care of this automatically.
- Test Your URL: Print the final URL (
response.url
) to verify the parameters are appended correctly before proceeding.
Advanced Usage
More complex scenarios might require additional functionality, such as handling headers, POST parameters, or authentication tokens. Here’s an example that demonstrates combining URL parameters with headers:
import requests
url = "https://api.example.com/resource"
parameters = {
"search": "python programming",
"page": 2
}
headers = {
"Authorization": "Bearer your_access_token_here"
}
response = requests.get(url, params=parameters, headers=headers)
print("Final URL:", response.url)
print("Response:", response.json())
In this case, the headers
dictionary adds an authentication token to the request while the params
dictionary handles the URL parameters.
Error Handling
When working with API requests, always account for the possibility of errors. Use status codes and exception handling to make your code more robust. For example:
try:
response = requests.get(url, params=parameters)
if response.status_code == 200:
print("Success:", response.json())
else:
print("Error:", response.status_code)
except requests.exceptions.RequestException as e:
print("Request failed:", e)
Conclusion
Passing parameters into URLs for Python requests is a straightforward process once you understand the basics. The library’s built-in capabilities handle much of the heavy lifting, such as encoding parameters and handling special characters. By organizing your parameters effectively and testing your URLs, you can minimize errors while maximizing the efficiency of your code.
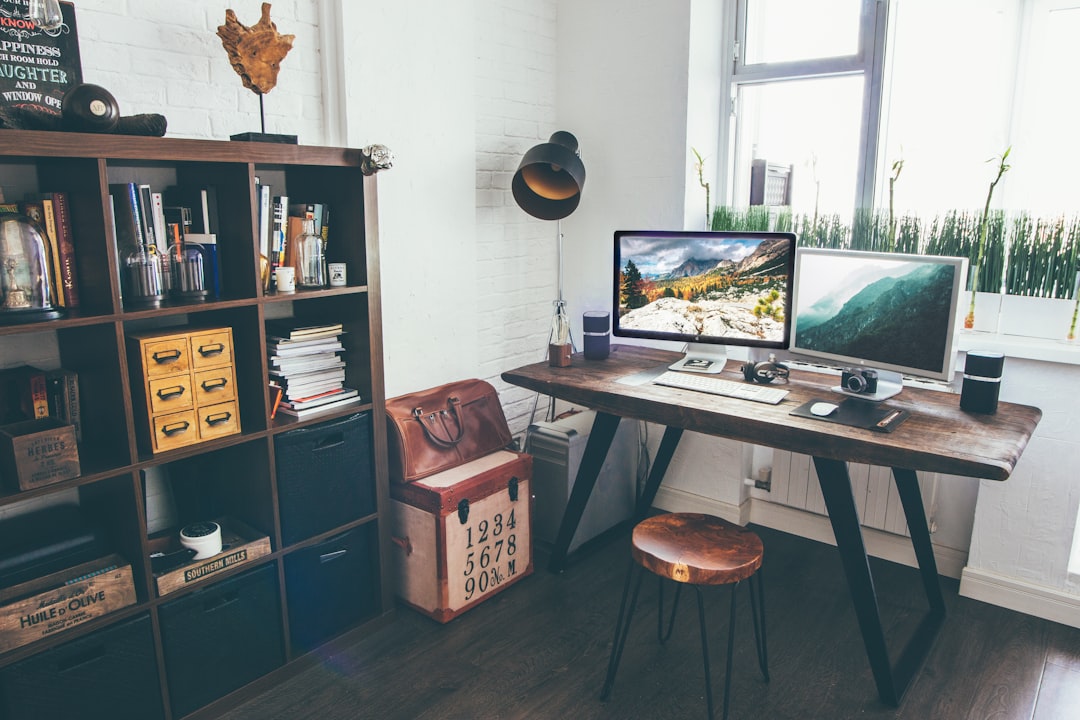
Whether you’re a beginner learning to make API requests or an advanced developer working on complex integrations, mastering URL parameters is an indispensable skill for any Python programmer.